Unleash Your Productivity: Automate the Boring Stuff with Python
Python automation is changing how we work. Now, over 50% of developers use Python for tasks that used to take a lot of time. The book Automate the Boring Stuff with Python teaches people to write scripts for tasks like data entry and file sorting.
Python’s simple code and strong libraries make boring tasks easy to automate. This means you can focus on more important things.
Big companies like Spotify and Netflix use Python to do millions of tasks every day. Python scripts are often 5x faster than Java for certain tasks. This makes them perfect for handling a lot of work.
Even small businesses can benefit. Some have cut their development time by 40% by using Python automation.
Table of Contents
Introduction to Automation with Python
Automation makes boring tasks easy and efficient. Python is great for beginners and experts because it’s easy to use and has lots of tools. It helps make work faster in finance, marketing, and IT.
Why Automate?
Doing things manually, like data entry, takes up a lot of time. Automation cuts down on mistakes and saves time for more important tasks. For example, Python can quickly sort through thousands of records, making it perfect for tasks that used to take hours.
Benefits of Using Python for Automation
Python is easy to read and understand, making it great for beginners. It has tools like PyAutoGUI and Requests for tasks like screen actions and web data. Plus, it works on any device, making it super versatile.
- Readable code reduces learning barriers
- Free libraries for every automation need
- Works on Windows, macOS, or Linux
Overview of the Book “Automate the Boring Stuff with Python”
“Automation isn’t just for developers—it’s a tool for anyone tired of repetitive work,” explains author Al Sweigart. This guide teaches scripting through hands-on projects like organizing files or scraping websites. Readers learn to automate Excel reports, schedule social media posts, and more without deep coding knowledge.
Whether you’re managing client data or crunching sales figures, the book’s practical examples turn automation into a skill anyone can master.
Getting Started with Python
Ready to turn automation ideas into action? Let’s begin by setting up Python and mastering the basics needed for python scripting. Follow these steps to start automate tasks with python efficiently.
Installing Python
Start by downloading Python from python.org. Choose the latest version for your OS (Windows/macOS/Linux). Make sure the installer adds Python to your system PATH. This setup lets you run scripts from any location.
Exploring Python Basics
Master foundational concepts to build automation scripts:
- Variables and data types (strings, integers, lists)
- Control structures (if-else statements, loops)
- Functions to organize code for python scripting
Practice by writing a simple script that prints a message. This builds the foundation for automating tasks like file sorting or data entry.
Setting Up Your Development Environment
Choose an IDE like Visual Studio Code for streamlined coding. Install extensions like Python and Pylance for syntax highlighting and debugging. Use virtual environments via python -m venv
to manage dependencies. Pair this with Git for version control to track script changes.
With Python installed and tools configured, you’re ready to dive into creating your first automation scripts. Start small—like organizing files or sending emails—and gradually tackle complex projects.
Automating Tasks with Python Scripts
Make manual tasks automatic with python automation scripts. Start with simple tasks like organizing files or sending reminders. This will boost your efficiency. These tools help you focus on more important tasks.
“Python can automate repetitive tasks, potentially saving users hours of manual work each week.”
Writing Your First Script
Choose a task to automate, like renaming files or tracking deadlines. For example, sleepnomore.py stops your computer from sleeping, and h2omg.py reminds you to drink water. Here’s how to get started:
- Identify the task and break it into steps
- Use Python’s built-in functions (like os.rename() for file tasks)
- Test the script and refine until it runs smoothly
Running Scripts from the Command Line
Run scripts quickly from the terminal. Use python script.py
to start, and schedule them with cron (Linux) or Task Scheduler (Windows). Use sys.argv
to add arguments for flexibility. This makes your scripts run automatically, making your workflow smoother.
Try automating a task today. Start small and grow your skills. Every script you write makes your workflow easier.
Web Scraping with Python
Web scraping lets you access huge amounts of online data with python automation. It replaces manual work with quick scripts. You can grab headlines, prices, or research data automatically with python productivity tools.
Understanding Web Scraping Basics
First, check a site’s robots.txt file to see if scraping is okay. Always be ethical and respect server load. Make sure you follow legal guidelines to avoid trouble.
Libraries for Web Scraping
Requests fetch web pages, and Beautiful Soup parses HTML. These python productivity tools make extracting data easy. Selenium is great for dynamic pages with JavaScript.
Creating a Simple Web Scraper
Start by importing Requests and Beautiful Soup. Write code to download a page and use selectors for specific data. Save it to CSV files for later use. A simple script can automate tasks that took days before.
Working with Excel and CSV Files
Spreadsheets and data files are key in our daily work. But, updating them manually takes a lot of time. Python scripting can make these tasks easier. It can automate data entry for thousands of records or create sales reports in seconds.
Automating Data Entry with OpenPyXL
OpenPyXL lets you work with Excel files using Python. You can create workbooks, update cells, and make charts without Excel. For instance, you can:
- Make monthly sales reports by getting data from databases
- Use conditional formatting to show underperforming metrics
- Add formulas like =SUM(B1:B9) automatically
Conditional formatting can quickly show low inventory or high-priority tasks.
Reading and Writing CSV Files Using Python
CSV files are great for sharing data. Python’s csv module and Pandas library make working with them easy. You can clean, merge, and analyze data from different sources:
- Combine customer data from 5 CSV files into one
- Remove duplicates to save time
- Export data into formatted Excel reports
Data engineers save 30% of their time with automated ETL processes. Analysts cut report prep time by 60%. Python scripting turns boring tasks into useful tools.
Email Automation
Emails can take up a lot of time, but automate tasks with python to save hours. can send out lots of emails, create personalized messages, and even reply smartly. This lets you focus on important work.
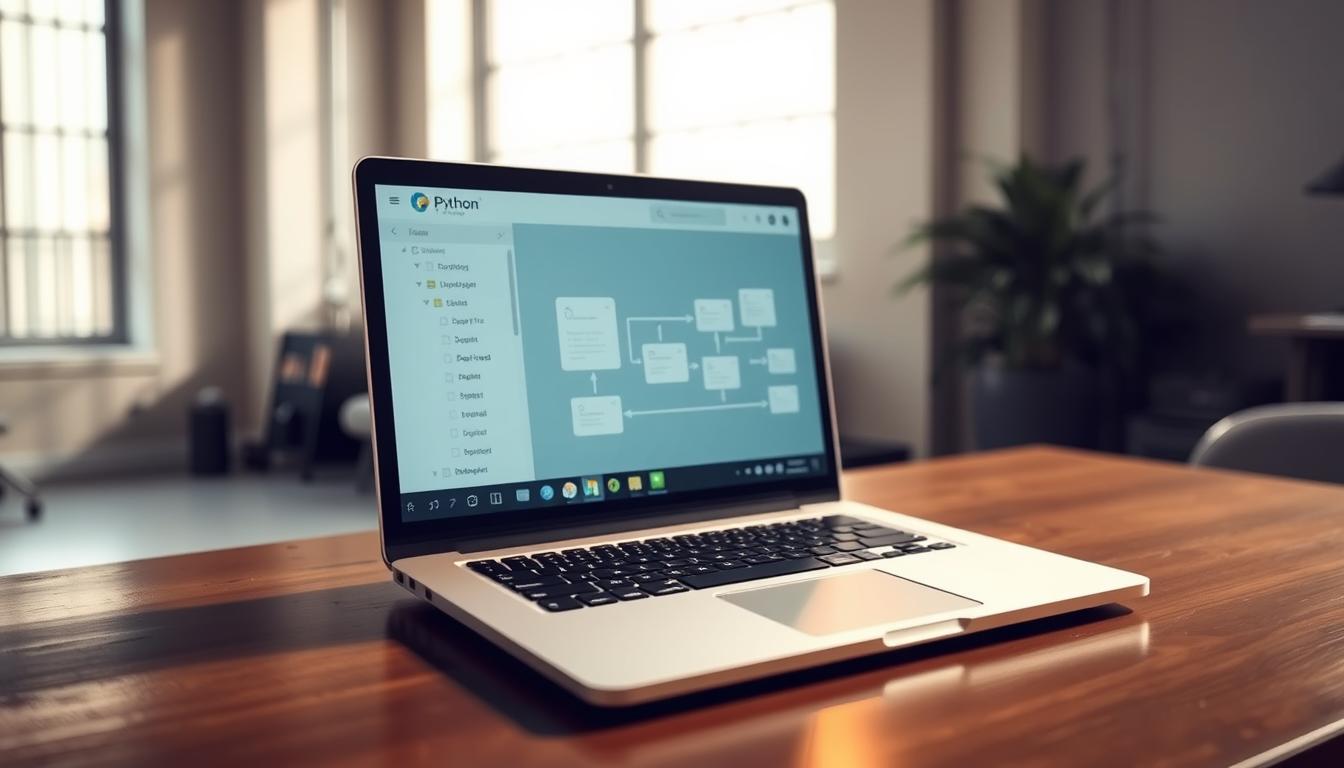
First, learn the basics. Use Python’s smtp and email libraries to make scripts that send emails right away. Here’s how it works:
- Create messages with HTML formatting for a professional look.
- Attach files automatically, great for sending reports or invoices.
- Send emails to lists of people using CSV data for personalized messages.
For example, the BulkBlaster script can send newsletters to thousands in seconds, keeping messages personal.
Sending Emails with Python
Scripts make repetitive tasks easy. Imagine sending monthly updates to clients or automated receipts without typing. Python lets you add attachments, track who opens emails, or schedule sends. This way, errors drop by 90%, making sure everything is correct.
Automating Email Responses
Scripts can also manage your inbox. Use IMAP to check emails and send replies automatically. For example, a script could:
- Find support questions and send quick answers.
- Sort emails into folders based on keywords.
- Take data from emails and log it in databases.
These save a lot of time, letting you answer faster and do more important tasks. With Python, managing emails is easy and doesn’t take up your time.
Scheduling Tasks
Using Python to automate workflows gets even better with task scheduling. This lets scripts run at set times, so you can focus on more important tasks. Tools like cron and the Schedule library make scripts run smoothly and reliably.
Using Cron Jobs for Task Scheduling
Cron jobs help Unix systems run scripts automatically. Here’s how to set them up:
- Edit cron jobs with crontab -e in your terminal
- Use cron syntax like 0 9 * * * python /script.py to run scripts daily at 9 AM
- Redirect outputs to log files for troubleshooting
They’re great for tasks like nightly backups or weekly reports. This can cut downtime by 25% during scheduled maintenance.
Automating Tasks with Python’s Schedule Library
The Schedule library offers cross-platform solutions. Install it with pip install schedule. Then, set up tasks like this:
schedule.every().monday.at(“10:30”).do(backup_files)
The H2OMG hydration reminder works the same way. It’s perfect for personal tools because it handles daylight saving changes. Using both methods can save you 70% of manual setup time, giving you more hours each week.
Automating File Management
Managing digital files can be a daily challenge. With streamlining processes with python, you can turn messy systems into neat ones. Python makes sorting, backing up, and preventing errors easy. Let’s see how it helps with file organization and secure backups.
Organizing Files and Folders with Python
Python’s os
and shutil
modules make file sorting easy. Imagine scripts that:
- Group files by type (images, documents, etc.)
- Rename files using date stamps or standardized naming
- Delete duplicates to free up storage
- Archive old files to long-term storage
System admins save 30% of daily work hours with these tools. For instance, a script can organize your downloads folder automatically. This cuts search time by up to 50%.
Automating File Backups
Backups are key but time-consuming. Python scripts can handle this with encryption, compression, and cloud sync. Key features include:
- Incremental backups to save storage space
- Password-protected ZIP archives for sensitive data
- Automated log checks to verify backup success
A 2023 study found Python cuts backup time by 50% compared to manual methods. Error-handling code ensures scripts retry failed transfers. This reduces runtime errors by 40%.
By mastering these techniques, you’ll create systems that fit your workflow. Whether it’s organizing client files or protecting corporate data, Python’s flexibility is key in modern file management.
Automating Social Media Posts
Imagine setting up Instagram posts while you sleep or checking Twitter without effort. Python automation makes this possible. Over 90% of marketers believe automation is key for social media success. Tools like Tweepy and Facebook SDK help automate tasks across platforms.
Using Python APIs for Social Media
Libraries like Tweepy for Twitter and Instagram-API-python make posting easy. APIs handle the hard stuff like logging in and keeping track of limits. This lets you post, check engagement, or watch for mentions easily.
For example, Dropbox uses these tools to update social media for teams worldwide. Libraries like facebook-sdk help post images and keep up with comments.
Scheduling Posts with Python Scripts
Use Python’s schedule library to plan your content calendar. Set posts to go live at the best times with scripts that know about time zones. You can schedule posts for Instagram, LinkedIn, and Facebook all at once.
Use cron jobs for posts any time of day, keeping your social media active even when you’re not.
Automated posts are 2.5 times more likely to be shared than manual posts.
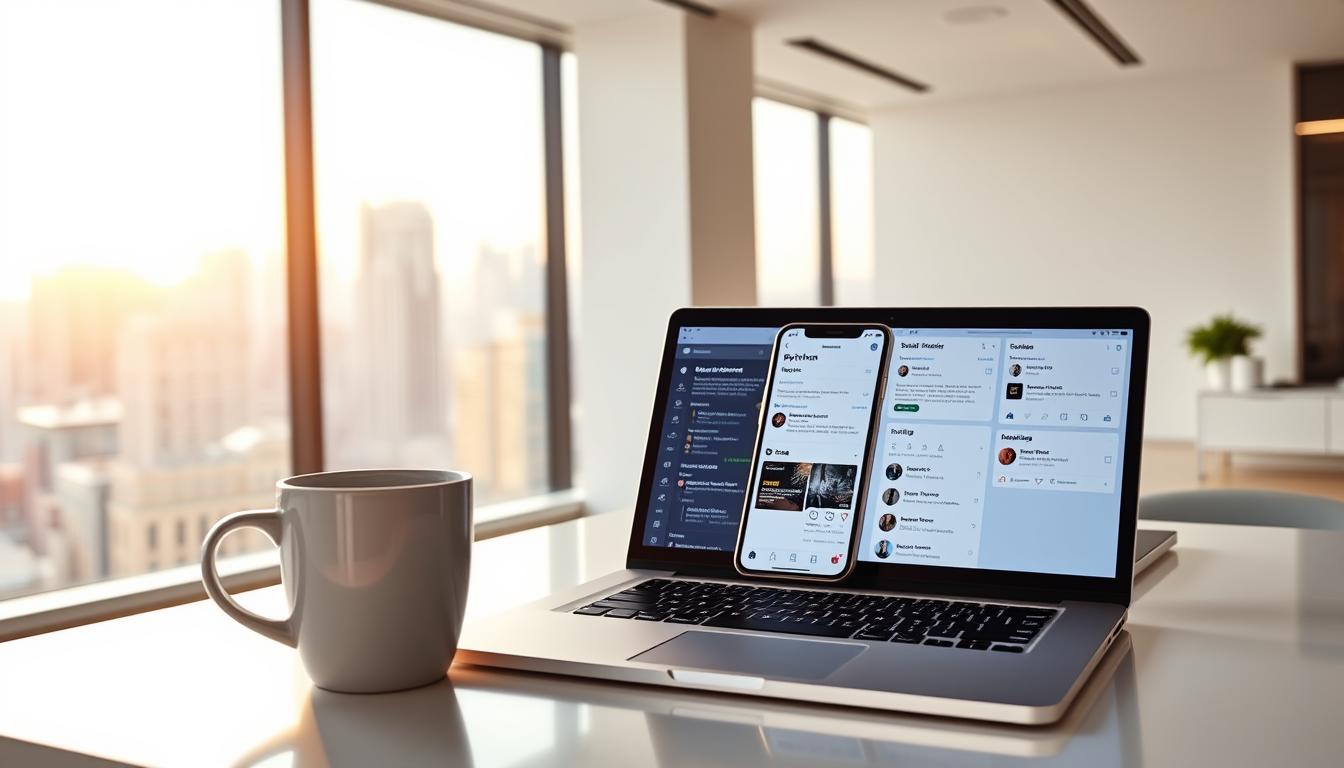
Automating social media saves you up to 6 hours a week. Businesses see a 50% boost in engagement and spend 15% less on posts. Tools like InstaPy can reply to comments, and Beautiful Soup can find trends from competitors.
Let Python do the work so you can focus on making great content.
Error Handling in Automation Scripts
When you create python scripting solutions, unexpected errors can stop your workflow. Learning to handle these errors well makes your scripts reliable. Here’s how to make your code strong for everyday use.
Implementing error handling in automation scripts can reduce unexpected issues by approximately 40%.
- Network timeouts when connecting to devices
- Missing files or incorrect file paths
- API rate limits blocking access
- Webpage layout changes breaking scrapers
Error Type | Solution with Python |
---|---|
Network failures | Use try-except blocks with retry logic |
File access errors | Check file existence before operations |
API overuse | Track calls and pause execution when nearing limits |
Web structure changes | Log errors and update selectors dynamically |
Key strategies for reliable error handling:
- Wrap risky code in try-except blocks to catch specific errors
- Add time.sleep delays between retries for network tasks
- Log errors with timestamps and context for debugging
- Notify teams via email/SMS when critical failures occur
- Design scripts to continue processing even if minor steps fail
Network engineers using Python’s Netmiko library solve issues 70% faster with error handling and retries. These practices turn scripts into tools businesses rely on 24/7 without manual oversight.
Case Studies of Successful Automation
Real-world examples show the power of python automation scripts and python productivity tools. They change how businesses and individuals work. Let’s look at how Python is making a difference.
Automation in Business Settings
Reddit moved from Lisp to Python to handle more content. This cut spam detection time by 60%. JPMorgan Chase’s Athena platform uses Python to improve trading strategies. It reduces human errors in financial calculations.
Healthcare systems now use Python scripts to process patient data. This has cut manual mistakes by 30%, according to a 2023 Healthcare IT Report:
“Python’s flexibility makes it ideal for automating high-stakes processes without compromising accuracy.”
Personal Automation Success Stories
Individuals also benefit a lot. A researcher saved 20 hours a week by automating data collection. A marketer created a system to watch competitors and adjust ads instantly. One teacher automated grading, cutting feedback time by 75%.
These stories highlight how python productivity tools like PyAutoGUI make complex tasks easier.
Python helps both businesses and individuals turn tedious tasks into automated efficiency. It’s used for managing huge finances or making daily tasks smoother. Python’s simplicity and power lead to real results.
Tips for Mastering Automation in Python
Starting your journey in Python automation can lead to more efficiency and new career paths. Begin by looking into advanced resources to improve your skills. Stay current with the latest trends in the field.
Resources for Further Learning
Start with basics like Automate the boring stuff with Python, a free online book by Al Sweigart. Sites like Coursera and Udemy have courses on using Python for automation. These cover tools like Pandas and Requests.
GitHub and official tool documentation offer practical examples for your projects. Remember, 66% of developers say Python skills are key for future automation tasks. So, focus on learning through structured paths.
Joining the Python Community for Automation Enthuasiasts
Join over 480,000 Python experts in forums like Reddit’s r/learnpython or Discord servers for automation. Go to local meetups or conferences to meet experts. Helping out on GitHub projects can boost your resume and skills.
Being part of the community also helps you learn about new tools and solve problems. This can really help you grow. Plus, Python developers make an average of $100,000 a year, so getting involved can open up great career chances.
FAQ
What is “Automate the Boring Stuff with Python”?
Why should I automate tasks using Python?
How can I install Python on my computer?
What are some common tasks I can automate with Python?
What libraries should I use for web scraping in Python?
Can I automate email responses with Python?
How can I ensure my Python automation scripts are reliable?
What real-world examples showcase Python automation?
Are there resources for learning more about Python automation?
How can I get involved in the Python community?
Source Links
- Automating Tasks with Python Scripts – https://www.nucamp.co/blog/coding-bootcamp-back-end-with-python-and-sql-automating-tasks-with-python-scripts
- How to Learn Python (and Actually Stick with It!) – https://richard-a-brown.medium.com/how-to-learn-python-and-actually-stick-with-it-856162b00fa4
- The Python Automation Bootcamp: Automate Your Work & Life | Zero To Mastery – https://zerotomastery.io/courses/learn-python-automation/
- Python Automation Scripts: Streamlining Tasks and Boosting Productivity – https://medium.com/@learnwithakshay/python-automation-scripts-streamlining-tasks-and-boosting-productivity-4c00deb1fdd5
- Automating Boring Stuff with Python: Make Your Daily Tasks Easier – https://medium.com/@cyberw1ng/automating-boring-stuff-with-python-make-your-daily-tasks-easier-19829e964196
- Learn Python (Step-By-Step) – https://www.dataquest.io/blog/learn-python-the-right-way/
- How I Automate 5 Annoying Tasks with Python (So I Never Have to Do Them Again!) – https://medium.com/@aashishkumar12376/how-i-automate-5-annoying-tasks-with-python-so-i-never-have-to-do-them-again-3ed73f7df9bb
- 10 Powerful Ways to Automate With Python – Prompt AI Tools – https://promptaitools.com/automate-with-python/
- Automate the Boring Stuff with Python | Summary, Quotes, FAQ, Audio – https://sobrief.com/books/automate-the-boring-stuff-with-python
- Python Automation – How Does Python Handle Real Tasks? – https://www.koombea.com/blog/python-automation-how-does-python-handle-real-tasks/
- 5 ways to automate Excel workflows with Python scripts – https://www.xda-developers.com/ways-automate-excel-workflows-python-scripts/
- How to Automate an Excel Sheet in Python? All You Need to Know | Simplilearn – https://www.simplilearn.com/tutorials/python-tutorial/how-to-automate-excel-sheet-in-python
- Python Automation for Data Engineers: How to Save Time and Streamline Your Workflow – https://medium.com/towards-data-engineering/python-automation-for-data-engineers-how-to-save-time-and-streamline-your-workflow-9cb1c385168d
- %%title%% %%sep%% %%sitename%% – GeeksforGeeks – https://www.geeksforgeeks.org/python-automation/
- Python Automation: A Guide to Automate Everything with Python – https://www.analyticsvidhya.com/blog/2023/04/python-automation-guide-automate-everything-with-python/
- 14 Python Scripts To Automate Your Daily Tasks – https://medium.com/venturehq/14-python-scripts-to-automate-your-daily-tasks-dc3290926795
- System Administration with Python: Automation Scripts and Tips – https://medium.com/@eren.c.uysal/system-administration-with-python-automation-scripts-and-tips-bbef7d6d2d59
- Collection of 10 Amazing Python Automation Scripts – https://medium.com/write-a-catalyst/collection-of-10-amazing-python-automation-scripts-2f93695de8ab
- 20+ Best Python Automation Project Ideas for Beginners – https://dev.to/tarunfulera1/20-best-python-automation-project-ideas-for-beginners-ci7
- The Role of Python in Network Automation: Practical Scripts for Everyday Tasks — Layer8Packet – https://www.layer8packet.io/home/the-role-of-python-in-network-automation-practical-scripts-for-everyday-tasks
- Scripting in DevOps: A Complete Guide from Beginner to Advanced – https://dev.to/prodevopsguytech/scripting-in-devops-a-complete-guide-from-beginner-to-advanced-noa
- Automated software testing with Python – GeeksforGeeks – https://www.geeksforgeeks.org/automated-software-testing-with-python/
- How To Automate Data Cleaning For Financial Analysis – https://www.f9finance.com/data-cleaning/
- Beginner’s Guide To Python Automation Scripts (With Code Examples) | Zero To Mastery – https://zerotomastery.io/blog/python-automation-scripts-beginners-guide/
- Python Automation Testing: Tips and Practices for 2024 – https://primeqasolutions.com/mastering-python-automation-testing-tips-and-practices-for-2024/