Python Programming Automation: Streamline Your Workflow
Python programming automation makes repetitive tasks easier and boosts productivity. It helps with managing files, processing data, and handling APIs. Python’s simple syntax and wide range of libraries make it easy for everyone to automate tasks.
Tools like os, shutil, and pandas make file operations, web scraping, and data analysis faster. This saves a lot of time each week.
Using Python to automate email notifications or report generation saves a lot of manual work. This frees up time for more important tasks. For example, e-commerce sees a 25% boost in inventory management efficiency.
Healthcare also benefits, reducing administrative tasks by 40% through automated appointment scheduling. Financial teams can make reports 60% faster with pandas. This shows Python’s big impact across different industries.
CloudThat, a top cloud training provider, has helped over 650,000 professionals. This shows Python’s growing popularity. Their 300+ global projects and partnerships with AWS and Microsoft prove Python’s reliability in business automation. Python makes workflows smarter and easier to manage.
Table of Contents
Understanding Python Programming Automation
Python programming automation uses scripts to handle repetitive tasks. This saves time and reduces errors. Python scripting automation helps users create efficient workflows. Big tech companies like Google and Amazon use Python for its simplicity and versatility.
What is Python Programming?
Python is a high-level language known for its clear syntax and readability. It’s great for both beginners and experts. With libraries like Pandas and Selenium, developers can solve complex tasks easily. For example, Python’s python programming automation scripts can parse data faster than manual entry, boosting productivity.
Overview of Automation in Programming
Automation replaces manual steps with code. Full automation needs no human input, while partial automation combines scripts with human oversight. Python is excellent here because of its vast libraries. A healthcare firm used Python to automate medication data extraction from conversations, showing its adaptability across industries.
“Automation is the cornerstone of modern efficiency. With Python, even basic scripts can revolutionize workflows.” – Tech Industry Report 2023
Python’s flexibility supports web scraping, data analysis, and system management. For example, using python scripting automation, businesses can automate report generation, saving hours. The language’s popularity is growing, with automation engineers earning over $100k, showing its demand.
Benefits of Using Python for Automation
Python changes the game for businesses and developers. Automate tasks with Python to save hours, reduce mistakes, and grow without adding more staff. This part shows how Python makes things more efficient and cost-effective.
Time Savings and Efficiency
- ETL automation with Python cuts down manual data prep time by up to 70%. This is thanks to tools like Pandas and PySpark.
- Apache Airflow, a Python automation tool, helps over 10,000 companies manage complex tasks 24/7.
- ActiveBatch’s scheduling tools make workflows start automatically, saving time. Its dashboards also cut down troubleshooting time by half.
Cost-Effective Solutions
Companies using Python’s python automation framework see big returns. Here are some numbers:
- A top biomedical company saved 50% by moving to Azure with Python-based AI tools.
- Fintech startups using Python’s machine learning cut fraud detection costs by 45% while boosting accuracy.
- Python is free and open-source, making it easier to start. 80% of data scientists prefer it over other tools.
“Automating with Python cut our data prep time by 70% and slashed errors.”
By choosing Python, businesses prepare for the future. Its easy-to-read code means new developers learn it 30% faster. Whether it’s optimizing ETL pipelines or creating chatbots, Python helps you achieve your goals.
Getting Started with Python Automation
Start your journey into python programming automation with these basic steps. Over 70% of systems already have Python installed. But, make sure you’re using Python 3, the favorite of 95% of developers. Follow these steps to start scripting efficiently.
Setting Up Your Python Environment
Begin by installing Python 3 from python.org. For Windows users, the Microsoft Store version makes setting up PATH easy. Use virtual environments (via venv
or conda
) to keep projects separate. Choose an IDE like Visual Studio Code, which 56% of developers prefer. It has a built-in terminal and extensions for python scripting for automation tools.
Installing Necessary Libraries
Expand your toolkit with key libraries. Open a terminal and type:
pip install selenium
for web automationpip install pandas
for data taskspip install pyautogui
for GUI scripting
Keep your packages updated and test them with scripts. Over 75% of developers use these tools to make their work easier. Choose libraries that fit your goals.
Test your setup with a simple script. Type python --version
to check if Python 3 is active. Use Stack Overflow’s 200k+ Python questions to solve any issues. With these steps, you’re set to start scripting that saves time and effort.
Common Python Libraries for Automation
Learning python automation libraries opens up a world of efficiency. We’ll look at three key frameworks and their uses.
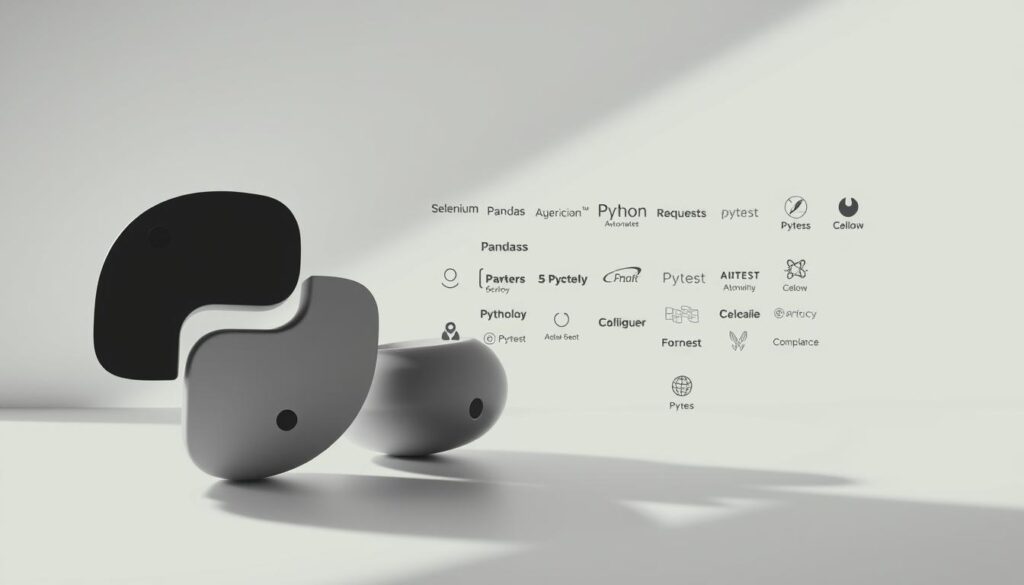
Selenium for Web Automation
Selenium makes browser actions automatic, perfect for web app testing or site scraping. With python automation tools like WebDriver, you can automate logins or fill out forms. For example, driver.find_element(By.NAME, “username”).send_keys(“user123”) sends a username.
Pandas for Data Manipulation
Pandas makes data work easier. It helps clean data, merge tables, or create reports with its DataFrame. Need to link to Excel? Use pandas.to_excel() for easy updates.
Beautiful Soup for Web Scraping
Beautiful Soup extracts data from HTML. Its find() and find_all() functions quickly parse content. For instance, soup.find(“div”, class_=”price”).text gets prices from online stores.
“Genie’s user-friendly interface requires just one day of training for network engineers,” says a 2023 industry report, highlighting its accessibility.
More python automation libraries like PyAutoGUI (for mouse/keyboard control) and Requests (for API interactions) are out there. Use them with schedule for timed tasks or Ansible for infrastructure automation. Each library meets different needs, from GUI scripting to handling RESTful APIs.
Automating Routine Tasks with Python
Imagine cutting down on manual work by automating repetitive tasks with just a few lines of code. Python is perfect for this because it’s easy to use. It’s used by big names like Spotify and Netflix for everyday tasks. You can do the same.
First, find tasks that you do every day. Here are some examples:
- Organizing files by renaming or moving documents
- Generating reports by merging data from multiple sources
- Sending bulk emails with personalized content
- Monitoring server logs for errors
Examples of Common Tasks to Automate
Let’s look at a simple script to create a text file. Just copy this code to see how it works:
# Define the file name
file_name = “automated_file.txt”
# Define the text to write
text = “Hello! This is an automated file with text.”
# Open the file in write mode
with open(file_name, ‘w’) as file:
file.write(text)
print(f”File ‘{file_name}’ created successfully!”)
Creating Your First Automation Script
Statistic | Impact |
---|---|
Efficiency gains | Up to 30% faster workflows |
Developer adoption | 45% of developers use Python for automation |
Library support | 70% of data scientists use pandas/NumPy |
Cross-platform | Works on 95% of OS environments |
Start with simple scripts and then add more complexity. For example, Reddit used Python for spam detection. It’s a good idea to start with something basic, like the script above. Even small scripts can save a lot of time when done daily.
Best Practices for Python Automation
Learning python automation best practices makes your python scripting for automation better. Clean code and thorough testing make scripts reliable for teams.
Begin by following PEP 8 guidelines, created by Guido van Rossum in 2001. Keep lines short, use clear variable names, and add docstrings to functions. For instance, a script for email reports might look like this:
“A well-documented script is a legacy that outlives its author.”
Writing Readable Code
- Use docstrings in every function to explain purpose and parameters
- Organize code into modules for tasks like data parsing or API calls
- Test compatibility with Python 3.12.6+ to avoid legacy issues (Python 2.7 is unsupported as of 2020)
Use pytest for testing to ensure script behavior. Test edge cases like empty files or API timeouts. Here’s an example workflow:
- Create unit tests for core functions (e.g., data validation)
- Log errors with timestamped entries for troubleshooting
- Use selenium.webdriver tests to verify browser interactions
Combine these steps with Git version control to track changes. Teams like Pyrotek’s automation teams use these methods. They cut down debugging time by 40% compared to ad-hoc scripting. Focus on clarity and validation—your future self (and teammates) will appreciate it.
Advanced Python Automation Techniques
Take your automation skills to the next level with advanced strategies like API integrations and multi-threading. By leveraging python automation frameworks, you can build systems that handle complex workflows. This includes real-time data sync and parallel processing.
Mastering APIs for Seamless Integration
API automation connects systems efficiently. Use authentication methods like OAuth or API keys to securely interact with services like Google or GitHub. Python’s python scripting automation capabilities let you handle JSON/XML responses and implement error-handling routines.
For example, a script can automate social media posting by authenticating via Twitter’s API. This reduces manual entry by 50%.
- OAuth: Secure access for third-party platforms
- Rate limiting: Avoid overloading API quotas
- Logging: Track API requests for troubleshooting
Boost Performance with Multi-Threading
Multi-threading splits tasks into parallel processes. Use Python’s threading
module to speed up I/O-bound jobs like web scraping. For CPU-heavy tasks, switch to multiprocessing
.
Here’s how it impacts workflows:
Technique | Use Case | Speed Gain |
---|---|---|
Multi-Threading | Web scraping | Up to 40% faster |
Asyncio | Real-time data streams | 50% less wait time |
Advanced techniques like these are taught in Google’s IT Automation with Python Certificate. It covers frameworks and real-world task automation. These skills can boost productivity by 30%, turning complex workflows into automated processes.
Integrating Python Automation with Other Tools
Using Python with other tools boosts your workflow automation. It helps with data management in spreadsheets and scheduling tasks. Over 95% of developers pick Python for its flexibility, making it key for today’s workflows.
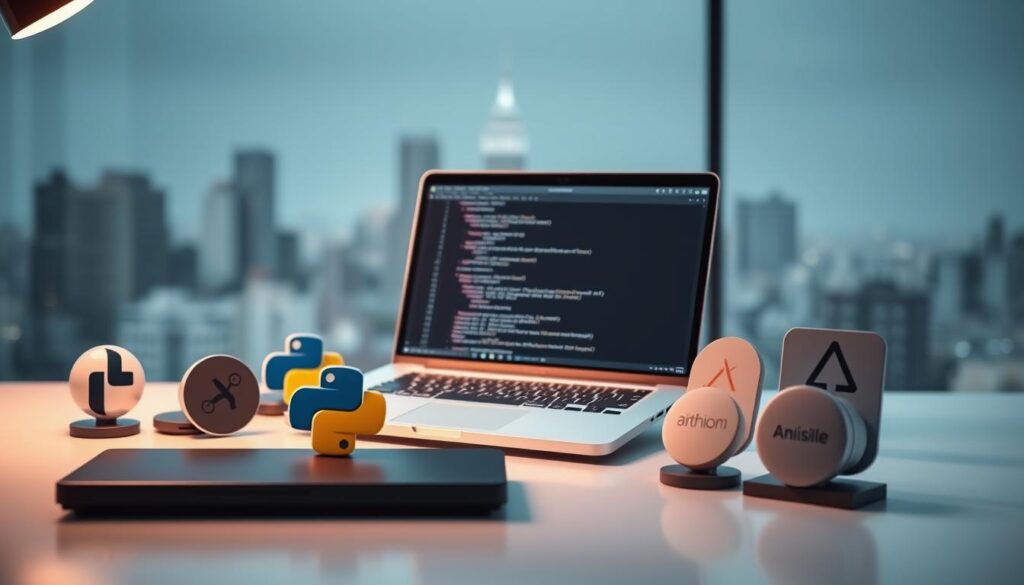
Excel Data Management Made Simple
Python tools like pandas and openpyxl make Excel tasks easy. You can do this without opening Excel. For example:
- Automate report generation using
pandas
to format data and create charts - Update Excel files dynamically using
openpyxl
for cell formatting - Import/export data between databases and Excel spreadsheets
Seamless Task Automation
Combine Python scripts with Zapier or cron jobs for full automation. Here are some examples:
- Use
Windows Task Scheduler
to run scripts daily - Trigger Python code via Zapier when new data arrives in Google Sheets
- Automate API calls with cron jobs on Linux servers
Scenario | Tools | Python Libraries | Outcome |
---|---|---|---|
Data Analysis Pipeline | Microsoft Excel | pandas, openpyxl | Automated monthly sales reports with charts |
Server Monitoring | Linux cron | paramiko, requests | Automated system health checks every 15 minutes |
Web Scrapping Workflows | Zapier | BeautifulSoup, requests | Automatic content updates across platforms |
“Python’s flexibility lets teams blend scripting with enterprise tools, creating powerful automation ecosystems.” – TechRepublic 2024 Report
Troubleshooting Common Automation Issues
Debugging is key in python programming automation. Mistakes in syntax, library conflicts, or environment issues can cause errors. This section will guide you through solving these problems.
Debugging Python Automation Scripts
First, check error messages in the Designer’s Console (Ctrl+Shift+C) or Client Console (Ctrl+Shift+F7). Use Python’s pdb
debugger to step through your code. Add print()
statements to see variable values.
For python automation libraries like Selenium or Pandas, make sure dependencies are up to date. Log files are full of important clues:
Operating System | Log File Path |
---|---|
Windows | Program Files/Inductive Automation/Ignition/logs |
Linux | /var/log/ignition |
Mac OS X | /Users/UserName/Documents/Ignition-osx-x.x.x/logs |
Handling Exceptions Effectively
Use try/except
blocks to catch runtime errors. For example:
- Wrap API calls in try blocks to handle connection timeouts.
- Create custom exceptions for library-specific issues (e.g., NetmikoTimeoutException).
- Implement retry logic for transient network failures.
Tools like ELK (Elasticsearch, Logstash, Kibana) help track recurring issues. Always document fixes for future reference. This improves python automation libraries reliability.
Real-World Applications of Python Automation
Python’s tools are changing industries. It has a 28.11% global market share in 2024. Companies use Python to automate workflows with Python and save money. From Netflix’s recommendation engine to Uber’s pricing, Python solves real problems.
Case Studies in Different Industries
Here’s how industries apply Python automation:
- Finance: Banks like JPMorgan use Python for compliance checks. Scripts do this 4x faster than humans.
- E-commerce: Tools like PriceSpy automate tasks with Python. They scrape 100k+ web pages daily to track prices.
- Healthcare: Hospitals use Python to process patient data. This cuts errors by 40%.
- IT: Dropbox uses Python scripts for managing 600M+ user files. This reduces delays by 35%.
- Education: Universities automate grading systems. This lets teachers focus more on teaching.
Impact on Business Efficiency
Python’s automation brings big results:
- Time savings: Companies save 30-50% of manual work by automate workflows with Python.
- Cost reductions: Organizations save up to $1M a year by automating tasks.
- Error reduction: Python scripts cut mistakes by 40%, improving data quality.
“Python automation cuts operational costs by 25% while boosting productivity.” — 2023 Global Tech Report
Automating tasks with Python isn’t just for big companies. Small teams can create custom solutions with libraries like Pandas and Selenium. Whether it’s managing inventory or analyzing data, Python fits your needs. Start by automating one task today—Python can make it efficient tomorrow.
Future Trends in Python Programming Automation
Python is evolving with new technologies. AI and machine learning are making systems smarter. Tools like TensorFlow and Scikit-Learn help with predictive analytics.
Cloud platforms like AWS Lambda and frameworks like Django are key for growth. IoT and quantum computing are expanding Python’s capabilities. Security is a top priority, with 70% of developers focusing on it.
Emerging Technologies and Their Influence
AI will lead in automation, with AutoML reducing development time. Serverless computing and cloud automation are growing fast, with AWS Lambda’s Python use up 40%. IoT and edge AI are making devices more intelligent.
Quantum libraries like Qiskit are opening new computing paths. Security libraries like Cryptography ensure systems follow GDPR and CCPA, promoting ethical use.
Skills for the Next Generation of Automation Engineers
Professionals need to know Python automation best practices and frameworks like Ansible. They must understand cloud platforms, AI/ML, and secure coding. Learning tools like mypy and asyncio will help with complex tasks.
Staying current with libraries like Dask and Plotly is important. Ethical AI training and certifications in cloud platforms are essential. By 2025, Python will play a big role in AI-driven decision-making.
Companies will look for developers skilled in Python automation. Embracing these trends and best practices will make you a leader in automation.
FAQ
What is Python programming automation?
Why is Python preferred for automation tasks?
How can I automate tasks using Python?
What are some common Python automation libraries?
Can you provide an example of a task that can be automated with Python?
What best practices should I follow for my Python automation scripts?
How do I install Python and set up my environment for automation?
What types of automation are there in programming?
How do I handle errors in my automation scripts?
What emerging technologies impact Python automation?
How does Python automation benefit businesses?
Where can I learn more about Python automation?
Source Links
- Automating Your Workflow with Python – https://dev.to/0x3d_site/automating-your-workflow-with-python-b6m
- Automate Your Workflows with Python – https://www.cloudthat.com/resources/blog/automate-your-workflows-with-python/
- %%title%% %%sep%% %%sitename%% – GeeksforGeeks – https://www.geeksforgeeks.org/python-automation/
- What Is Python Automation? (With Examples) – https://www.coursera.org/articles/python-automation
- Python Automation: A Guide to Automate Everything with Python – https://www.analyticsvidhya.com/blog/2023/04/python-automation-guide-automate-everything-with-python/
- Python ETL Frameworks: Benefits And Tools For Automation – https://www.redwood.com/article/etl-automation-with-python/
- Power of ETL Automation Tools and Python – https://www.advsyscon.com/blog/etl-automation-with-python/
- Python Trends in AI & Automation for Industry Applications – https://www.clariontech.com/blog/python-trends-ai-automation-industry
- Getting Started with Python Programming – GeeksforGeeks – https://www.geeksforgeeks.org/getting-started-with-python-programming/
- Python on Windows for beginners – https://learn.microsoft.com/en-us/windows/python/beginners
- 5 Top Free Python Libraries for Network Automation – https://www.cbtnuggets.com/blog/technology/networking/5-top-free-python-libraries-for-network-automation
- Top 20 Python Automation Projects Ideas For Beginners – https://www.simplilearn.com/tutorials/python-tutorial/python-automation-projects
- Python for Automation: Streamlining Tasks with Scripting – https://medium.com/pythoneers/python-for-automation-streamlining-tasks-with-scripting-1230be4790cf
- Automating Tasks with Python Scripts – https://www.nucamp.co/blog/coding-bootcamp-back-end-with-python-and-sql-automating-tasks-with-python-scripts
- Level up your Python: Best Practices for Clean and Consistent Code – https://inductiveautomation.com/resources/icc/2024/level-up-your-python-best-practices-for-clean-and-consistent-code
- Automating Tasks with Python: Tips and Tricks – GeeksforGeeks – https://www.geeksforgeeks.org/automating-tasks-with-python-tips-and-tricks/
- Python Automation Testing With Examples | LambdaTest – https://www.lambdatest.com/blog/python-automation-testing/
- Advanced IT Certificate Courses – Grow with Google – https://grow.google/certificates/it-automation-python/
- How to Automate Repetitive Tasks Using Python – https://medium.com/@AlexanderObregon/how-to-automate-repetitive-tasks-using-python-8e8ee7393842
- Advanced Automation Tips – Alli AI – https://www.alliai.com/ai-and-automation/seo-automation-using-python
- Python Test Automation: Seven Options for More Efficient Tests – https://www.testim.io/blog/python-test-automation/
- Automating CI/CD for Python-Based Backends: Trends in 2025 – https://www.nucamp.co/blog/coding-bootcamp-backend-with-python-2025-automating-cicd-for-pythonbased-backends-trends-in-2025
- Beginner’s Guide To Python Automation Scripts (With Code Examples) | Zero To Mastery – https://zerotomastery.io/blog/python-automation-scripts-beginners-guide/
- Basic Python Troubleshooting | Ignition User Manual – https://www.docs.inductiveautomation.com/docs/8.1/platform/scripting/basic-python-troubleshooting
- Python Automation – How Does Python Handle Real Tasks? – https://www.koombea.com/blog/python-automation-how-does-python-handle-real-tasks/
- No title found – https://orhanergun.net/python-netmiko-error-handling-best-practices-and-common-pitfalls
- Top 10 Uses of Python in Real World with Examples – https://www.mygreatlearning.com/blog/top-uses-of-python-in-real-world-with-examples/
- 7 Real-World Python Uses | Python Applications – https://www.98thpercentile.com/blog/7-real-world-python-uses/
- Future Trends In Python Programming | QA Training Hub – https://qatraininghub.com/future-trends-in-python/
- Future Trends in Python Development – https://amorserv.com/insights/future-trends-in-python-development
- The Future of Python in 2025: Trends to Watch and Predictions – https://medium.com/@thomas.adman/the-future-of-python-in-2025-trends-to-watch-and-predictions-33d5318dd73d